It is very easy to get started with C programming, in fact, you don’t even need to set up an environment to run C code there are lots of online compilers available out there in which we can write and compile our code within no time.
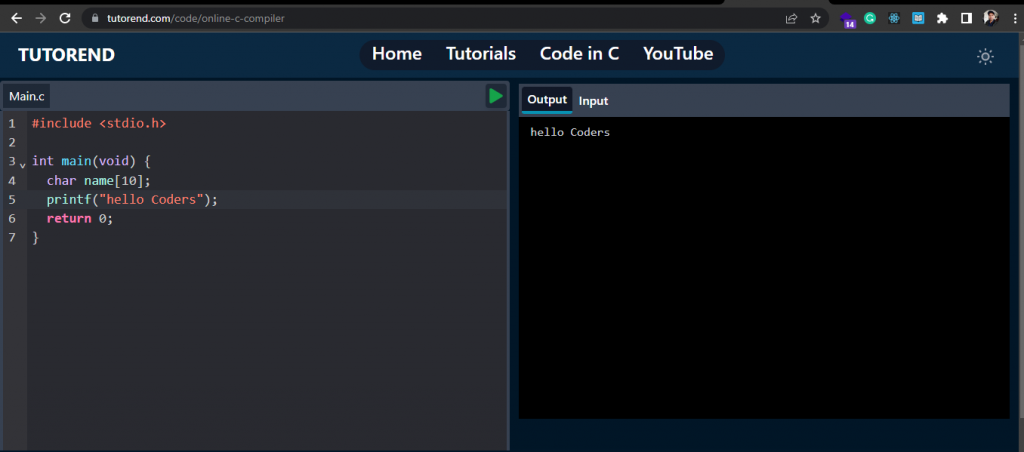
Tutorend itself provides you with an amazing Online C Compiler you can start from here but setting up a local environment is very important to learn the basics like how C compilers work and how our code is compiled.
#Basic C environment
You need two things in your computer before starting to code in C
- Text Editor – Whre you will write your C code, for example, Notepad
- C compiler – Which compiles our C code and outputs an executable program, for example, GCC
For Text Editor, you are good to go with the pre-installed Text editor Notepad if you are using Windows
If you want to set up a C environment this way then you can click on this link for step by step process.
#Using an IDE
An Ide or Integrated Development Environment is an all-in-one Text Editor which has advanced features you can write and compile code from only one window by clicking on some buttons.
Some of the Ide that supports C / C++ are Codeblocks, Visual Studio Code, Eclipse, etc.
#Setting Up Visual Studio Code for C / C++
Visual Studio Code (VS Code) is one of the most popular IDE and has lots of features like Emmet, Intelligence, Terminal, and Extensions.
Lots of developers choose Visual Studio code to write C / C++ code because of its error highlighting feature.
To setup VS Code for C / C++ we need three things
- Visual Studio Code
- C compiler ( MinGW )
- C / C++ extension for VS Code
#Installing Visual Studio Code
If you haven’t already installed Visual Studio Code then you can download it from this link https://code.visualstudio.com/Download
Download the version which suits best your Operating System and follow the instruction to install it.
#Installing MinGW
MingW is one of the easiest ways to install a C compiler on your computer, follow this link https://sourceforge.net/projects/mingw/ and download the installer which will help you to install a compiler for C, C++, and Fortran.
After downloading the MinGW installer, follow these steps to set up the C compiler:
- Run the downloaded installer and click “Install” on the welcome screen.
- Choose a directory where you want to install MinGW. It’s recommended to keep the default directory (C:\MinGW).
- Select the components you want to install. For this tutorial, you only need to select “mingw32-base” and “mingw32-gcc-g++” under “Basic Setup.”
- Click “Apply Changes” in the “Installation” menu.
- Wait for the installation to finish.
#Adding MinGW to PATH
To use the installed C compiler from any location in the command prompt, you need to add it to your system’s PATH variable. Follow these steps:
- Open the start menu and search for “Environment Variables.”
- Click on “Edit the system environment variables.”
- In the “System Properties” window, click on the “Environment Variables” button.
- Under “System Variables,” find and select the “Path” variable, then click “Edit.”
- Click “New” and add the path to the “bin” folder inside the MinGW installation directory (usually
- C:\MinGW\bin).
- Click “OK” on all the open windows to save the changes.
- Now you should be able to use the “GCC” command from the command prompt to compile C programs.
#Installing the C/C++ Extension for Visual Studio Code
To enable support for C/C++ in Visual Studio Code, you need to install the C/C++ extension provided by Microsoft. Follow these steps:
- Open Visual Studio Code.
- Click on the Extensions icon in the sidebar or press Ctrl+Shift+X to open the Extensions view.
- Search for “c++” in the search bar.
- Find the “C/C++” extension by Microsoft in the search results and click “Install.
Now that you have installed the necessary tools, you need to configure Visual Studio Code for C/C++ development. Follow these steps:
- Open Visual Studio Code.
- Create a new folder for your C project and open it in Visual Studio Code.
- Create a new file with a “.c” extension (e.g., “main.c”) to start writing your C program.
- Press Ctrl+Shift+P to open the Command Palette and type “C/C++: Edit Configurations (UI)” then press Enter.
- In the “C/C++ Configurations” tab, set the “Compiler path” to the path of the MinGW “gcc” executable (usually C:\MinGW\bin\gcc.exe).
- Save the settings and close the configuration tab.
- Now Visual Studio Code is configured for C development. You can write your C program, and the IDE will provide error highlighting, code completion, and other useful features.
To compile and run your C program, you can use the integrated terminal in Visual Studio Code:
- Press Ctrl+ to open the integrated terminal.
- Navigate to your project folder using the “cd” command.
- Compile your C program using the “gcc” command, followed by the input file name and the “-o” flag with the output file name (e.g., gcc main.c -o main).
- Run the compiled program by typing its name in the terminal (e.g., ./main on Linux/macOS or main on Windows).
Now you’re all set to start writing and running C programs using Visual Studio Code as your IDE.