Every programming language has a style of writing code or also called the structure of the program.
Similarly, C Programs also have a structure to follow while writing a program in it.
It’s the way program is supposed to be formatted and gets easily compiled and executed which if not followed may cause errors while compilation or runtime.
Below is the diagram showing different parts of the structure of a program.
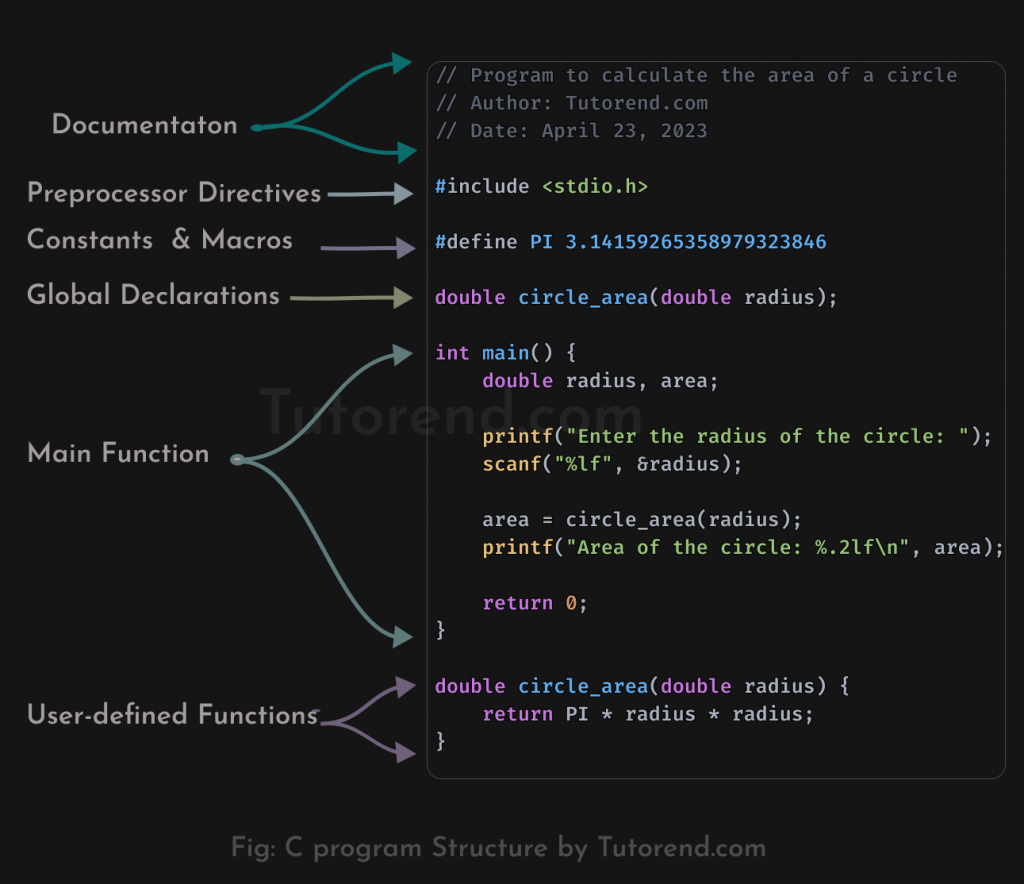
Let’s discuss each part separately.
#1. Documentation
// Program to calculate the area of a circle
// Author: Tutoren.com
// Date: April 23, 2023
Documentation in the c program also called comments is used to describe the program and its author, Comments are also used to describe what a specific part of the program is doing.
Comments are of two types.
#i. Single line comments
Uses //
to start and can be of only one line.
#include <stdio.h>
int main(void) {
//Single line comment
printf("Hello Coders!");
return 0;
}
#ii. Multi-line comments
Starts with /*
and ends with */
we can write multiple lines between the opening and closing of multiline comments.
#include <stdio.h>
int main(void) {
/*
Multi
line
comments
*/
printf("Hello Coders!");
return 0;
}
#2. Preprocessor Directives
// Preprocessor Section
#include <stdio.h>
To understand preprocessor directives let’s revise our vocabulary.
#Preprocessor
In the compilation process of a C program, the C program goes through 4 stages.
- Preprocessing: Expands our code with the meaning of directive commands like
#include
,#define
and removes comments. - Compiling: Compiles expanded code and generates assembly code.
- Assembly: Compiles generated assembly code into machine code ( binary instructions).
- Linking: Combines the object files and resolves external symbols and references and generates the executable program.
So the Preprocessor is the system program that usually comes with C compilers which is responsible for preprocessing.
Directive simply means giving instructions,
Preprocessor directives are commands for the preprocessors to perform certain operations, like including library code when we use #include
.
#3. Constants and Macros
// Macros and Constants
#define PI 3.14159265358979323846
This section mainly includes the constants and default values used throughout the program and uses #define
.
#4. Global Declarations & Function prototypes
// Function prototypes
double circle_area(double radius);
The global declaration means declaring some variable outside of the function on the top so that it can be accessed and manipulated from any function in the program without passing them as an argument.
Function prototypes are similar to global declaration where we declare a function name on the top but the actual definition of the function can be anywhere inside the program but still can be called from the function defined before it.
#5. Main Function
// Main Function
int main() {
double radius, area;
printf("Enter the radius of the circle: ");
scanf("%lf", &radius);
area = circle_area(radius);
printf("Area of the circle: %.2lf\n", area);
return 0;
}
The main function is the function from where every C program starts execution, all the logic is written here and variables are defined.
We can call any function (default/user-defined) inside our main function.
#6. User-defined functions
// User-defined Functions
double circle_area(double radius) {
return PI * radius * radius;
}
User-defined functions are the function that we as programs define in order to achieve our desired output.