When it comes to styling a website as quickly as possible, there is no good alternative to Bootstrap, Bootstrap gives us ready-made components to use inside our website and style it as quickly as possible.
While developing websites using REACT we have various ways to style our website and one of them is by using Bootstrap,
There are multiple ways of using Bootstrap in a REACT APP.
- Using Bootstrap from CDN
- Downloading compiled source files of Bootstrap.
- Using react-bootstrap package (specially created for react )
The prerequisite is to have a react app created, if not already created then use npx create-react-app my-app
to create a new react app if you have already created then continue.
#1. Using Bootstrap from CDN
Using Bootstrap from CDN is very simple you just have to include the CDN link of Bootstrap inside <head> </head>
tag inside the index.html file which you can find inside the public folder of your project and below is the code which you need to use.
<!-- CSS only -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0-beta1/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-0evHe/X+R7YkIZDRvuzKMRqM+OrBnVFBL6DOitfPri4tjfHxaWutUpFmBp4vmVor" crossorigin="anonymous">
<!-- JavaScript Bundle with Popper -->
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0-beta1/dist/js/bootstrap.bundle.min.js" integrity="sha384-pprn3073KE6tl6bjs2QrFaJGz5/SUsLqktiwsUTF55Jfv3qYSDhgCecCxMW52nD2" crossorigin="anonymous"></script>
after including both the script tag given above you’ll be able to use Bootstrap in your project right away.
#2. Downloading compiled Bootstrap source files.
Another method of using Bootstrap in React is also the same as using Bootstrap in any project that is the traditional way of downloading the source files of Bootstrap and linking them inside our HTML files and use as local CSS, JS files.
Follow this link to download Bootstrap 5.2 source files from the official website.
#3. Using React Bootstrap package
React Bootstrap is the special version of Bootstrap created especially to be used with React and is currently aligned with the Bootstrap version 5.1 don’t be confused with its own version which is currently 2.4.0.
You can follow this official documentation for better reference.
To use react-bootstrap we have to first install this package using below command
npm install react-bootstrap bootstrap
After the package is successfully installed now we have to import Components that we want to use from React Bootstrap.
For example, if we want to use the Butthon component of Bootstrap inside our project we have to import them as below
import "./styles.css";
import Button from 'react-bootstrap/Button';
//or
import { Button } from 'react-bootstrap';
export default function App() {
return (
<div className="App">
<h1> Hello Coders!</h1>
</div>
);
}
Now we are ready to use Button component inside our app like
import "./styles.css";
import Button from 'react-bootstrap/Button';
export default function App() {
return (
<div className="App">
<h1> Hello Coders!</h1>
<Button >Click me </Button>
</div>
);
}
Now when you refresh your app it should look like this
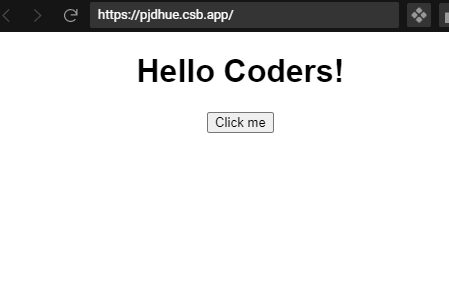
But as you can see there is no styling in the Button component, this is because React Bootstrap does not offer CSS styling unless we import it explicitly with
import 'bootstrap/dist/css/bootstrap.min.css';
and now your code should work fine and you can use every Component in similar way
To learn about more react-bootstrap Components Click Here .
That’s it for this tutorials hope you will like other tutorials too.