Using Icons on a website makes it look so beautiful and easy to navigate, and if we are building one with React Js or any React-based frameworks like Next Js we can use react-icons ( Icons library for react ) to import and use icons from hundreds of icons like Font Awesome 5, Ant Design Icons, Dev Icons, etc.
React Icons uses ES6-based import so we can choose to import only the icons we want to use rather than importing the whole library.
So let’s not waste your time and see how we can use it in our React or Next Js project.
#Installing react-icons
To use react-icons we have to install the NPM package of react-icons in our project.
Below is the command to add this package in your application.
npm install react-icons --save
Or, if you are using yarn use the following
yarn add react-icons
#Using Icons in our app
As we have installed the react-icons in our application, now we can import and use any of the icons from react-icons.
To see which icons are available you can visit this official website which has the catalog of all the icons available.
For example, I want to use a Hamburger icon from Font Awesome. So for that, there’s an icon named FaBars in react-icons and we can import it like below.
import { FaBars } from 'react-icons/fa';
Can you notice how we import the specific icon from the specific part of the library react-icons, ie. we are just importing FaBars
from fa
or a font awesome without importing the whole react-icons library, similarly, you can import any icon from the list.
Now we can use it as a component in our app like below
<FaBars />
import "./styles.css";
import { FaBars } from "react-icons/fa";
export default function App() {
return (
<div className="App">
<h1>Hello Coders!</h1>
<FaBars />
</div>
);
}
and you will get this output
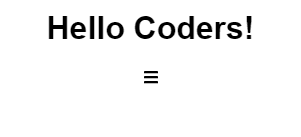
#Changing icon’s style
We can also change the style of the icons like size, color, and background color by passing some props.
<FaBars fontSize={40} />
this will increase the size of the icon and will display this
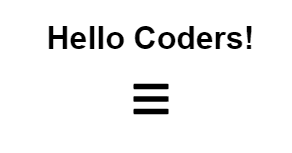
We can also style icons with CSS by utilizing className
<FaBars fontSize={40} className="my-icon" />
.my-icon{
color: blue;
background-color: yellow;
}
this will output
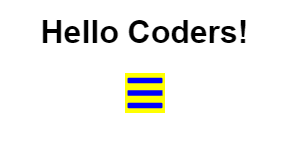
#Conclusion
Overall react-icons is a great package to solve all our icon needs in application development, it is also the most popular among React developers.
Finally, this is the full code of this tutorial which you can use as a reference at any time.
Well, you seem to be a patient programmer, I wish you never get a bug 😊 Happy coding.