Sharing feature on the website is very helpful for users to share the content with their friends and family. It is also helpful for website owners to get more traffic on their websites. It is very easy to implement sharing feature if you are building a website with React js or Next js. In this article, we will be using the react-share library to implement the social sharing feature in React or Next js.
react-share is a lightweight library that provides social sharing buttons for React js and Next js. It supports all the major social media platforms like Facebook, Twitter, LinkedIn, Pinterest, Reddit, Tumblr, Telegram, VK, Whatsapp, Line, and many more
Follow the steps below to implement the social sharing feature in React or Next js and if you prefer to watch a video tutorial then you can watch the above video.
#Project Setup
You can use react-share in your existing react or next js project or you can create a new project using create-react-app or create-next-app. I will be using codesandbox to create a new React project for this tutorial.
Here’s what my project looked like initially.
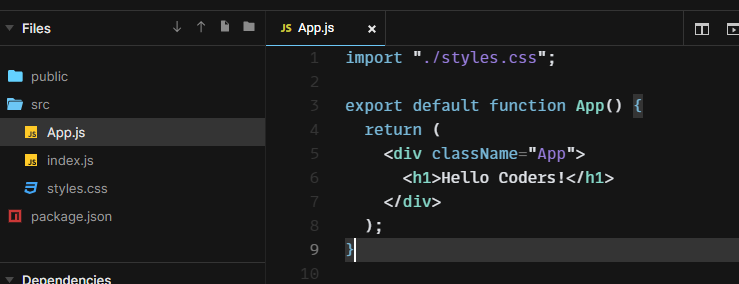
#Installing react-share
Now we can install react-share to use it in our project.
Run the following command in your terminal, and make sure that the terminal is open in the project directory.
npm install react-share
or if you are using yarn then run the following command
yarn add react-share
#Importing the required share buttons
Now open the component or page that you want to be sharable, in most cases it will be the page that contains the content like a blog post, in that case, open the page that renders the posts.
But in this tutorial, I am going to make our App
component sharable.
So in App.js
we are going to impost the share buttons from react-share.
There are enough of these buttons for most social media platforms, below is the list of all available buttons as of now.
EmailShareButton,
FacebookShareButton,
HatenaShareButton,
InstapaperShareButton,
LineShareButton,
LinkedinShareButton,
LivejournalShareButton,
MailruShareButton,
OKShareButton,
PinterestShareButton,
PocketShareButton,
RedditShareButton,
TelegramShareButton,
TumblrShareButton,
TwitterShareButton,
ViberShareButton,
VKShareButton,
WhatsappShareButton,
WorkplaceShareButton
For now let’s try to add share buttons for Facebook, Twitter, and WhatsApp.
import { FacebookShareButton, TwitterShareButton, WhatsappShareButton } from 'react-share';
#Creating Share Button
Every react-share components take at least one props url
and children.
<FacebookShareButton url={shareUrl}>
Share on Facebook
</FacebookShareButton>
And every component also has some additional props specific to them and some of them are optional.
Examples:
To pass the title in the share button we should give quota={title}
in FacebookShareButton while in TwitterShareButton we can pass title={title}
.
#Let’s prepare our sharable date
So when we think of sharing a page that means we will share the link of that page i.e, url
. You can get the URL of the page in many ways or you can just pass a static string if you want to share only one page.
If you want to get a dynamic URL then you can use the below method
// Get the URL of the current page dynamically
const shareUrl = typeof window !== 'undefined' ? window.location.href : '';
To get the Title dynamically you can use this
// Get the Title of the current page dynamically
const title = typeof document !== 'undefined' ? document.title : 'Check out this awesome content!';
Now as our URL and Title are ready we can pass them to share buttons.
#Use share buttons
Whatever share button you want in your app make sure that it is imported from react-share.
Here I am using Share buttons for Facebook, Twitter, and WhatsApp.
<div>
<FacebookShareButton url={shareUrl} quote={title}>
Share on Facebook
</FacebookShareButton>
<TwitterShareButton url={shareUrl} title={title}>
Share on Twitter
</TwitterShareButton>
<WhatsappShareButton url={shareUrl} title={title}>
Share on WhatsApp
</WhatsappShareButton>
</div>
Below is how my App.js looks now
import "./styles.css";
import {
FacebookShareButton,
TwitterShareButton,
WhatsappShareButton
} from "react-share";
export default function App() {
// Get the URL and title of the current page dynamically
const shareUrl = typeof window !== "undefined" ? window.location.href : "";
const title =
typeof document !== "undefined"
? document.title
: "Check out this awesome content!";
return (
<div className="App">
<div className="main">
<h1>Hello Coders!</h1>
</div>
<div className="share-buttons">
<FacebookShareButton url={shareUrl} quote={title}>
Share on Facebook
</FacebookShareButton>
<TwitterShareButton url={shareUrl} title={title}>
Share on Twitter
</TwitterShareButton>
<WhatsappShareButton url={shareUrl} title={title}>
Share on WhatsApp
</WhatsappShareButton>
</div>
</div>
);
}
Style.css
.App {
font-family: sans-serif;
text-align: center;
}
.share-buttons{
margin: auto;
color:blue;
display: inline-flex;
gap: 9px;
}
Let’s test the Output
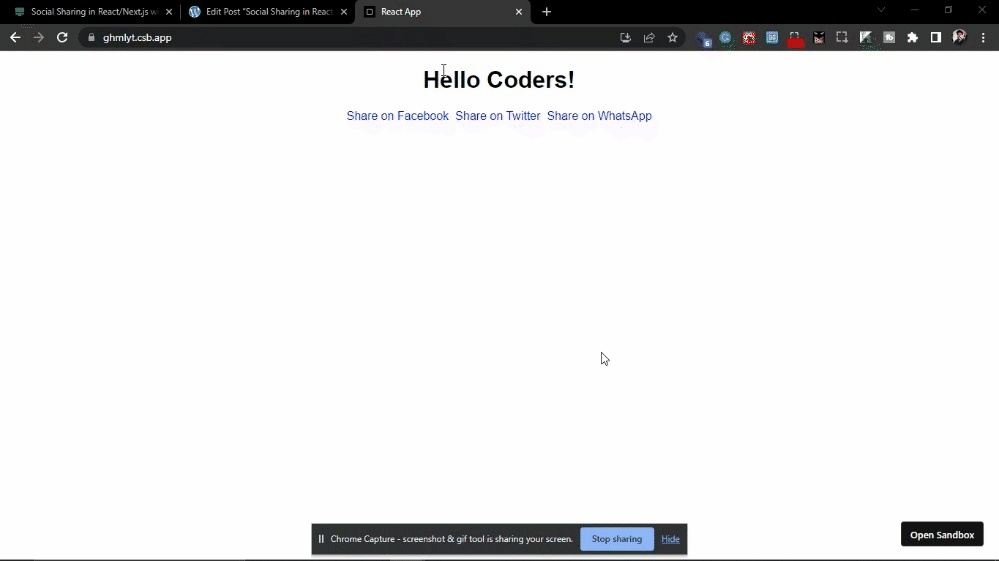
#Appearance
Till now we are only showing some text that is clickable to share the page but you can wrap any type of element that is valid as react node, also you can customize them however you want with the help of CSS.
For now, let’s just use some icons which are provided by the react-share library itself.
Below is the list of all the available when I am writing this but you can always check them on the official documentation.
import {
EmailIcon,
FacebookIcon,
FacebookMessengerIcon,
HatenaIcon,
InstapaperIcon,
LineIcon,
LinkedinIcon,
LivejournalIcon,
MailruIcon,
OKIcon,
PinterestIcon,
PocketIcon,
RedditIcon,
TelegramIcon,
TumblrIcon,
TwitterIcon,
ViberIcon,
VKIcon,
WeiboIcon,
WhatsappIcon,
WorkplaceIcon
} from "react-share";
and you can customize these icons by passing the following props
Props:
size: Icon size in pixels (number)
round: Whether to show round or rect icons (bool)
borderRadius: Allow rounded corners if using rect icons (number)
bgStyle: customize background style, e.g. fill (object)
iconFillColor: customize icon fill color (string, default = ‘white’)
So let’s use icons from this for our application, I am going to use FacebookIcon
, TwitterIcon
and WhatsappIcon
.
This is our updated code
import "./styles.css";
import {
FacebookShareButton,
TwitterShareButton,
WhatsappShareButton,
FacebookIcon,
TwitterIcon ,
WhatsappIcon
} from "react-share";
export default function App() {
// Get the URL and title of the current page dynamically
const shareUrl = typeof window !== "undefined" ? window.location.href : "";
const title =
typeof document !== "undefined"
? document.title
: "Check out this awesome content!";
return (
<div className="App">
<div className="main">
<h1>Hello Coders!</h1>
</div>
<div className="share-buttons">
<FacebookShareButton url={shareUrl} quote={title}>
<FacebookIcon size={50} />
</FacebookShareButton>
<TwitterShareButton url={shareUrl} title={title}>
<TwitterIcon round />
</TwitterShareButton>
<WhatsappShareButton url={shareUrl} title={title}>
<WhatsappIcon iconFillColor="pink"/>
</WhatsappShareButton>
</div>
</div>
);
}
And this is how our application looks now
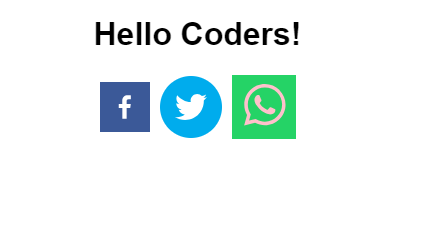
In the first icon, I have set size={5
0}
The second icon round
The third icon iconFillColor="pink"
#Conclusion
Overall react-share is a great combo of sharing features and icons for creating sharing features on a react or next js website and you can always customize the look and behavior of each icon and share button with props and CSS.
You can visit the official page for more information.
Open this codesandbox to view the live demo.
I think that’s enough demonstration and explanation to help you get started using react-share and I hope you enjoyed the tutorial.