Feather Icons is a collection of sleek and beautiful icons that you can use in your projects in different ways and integrate seamlessly with React-based applications.
To use it in our Next js application or React based applications we can use a package called react-feather
.
react-feather
is a collection of simply beautiful open-source icons for React.js. Each icon is designed on a 24×24 grid with an emphasis on simplicity, consistency, and readability.
Now let’s see how we can use React Feather Icons in our Next js applications, the process would be similar for React and other React-based frameworks.
#Step 1: Project Setup
I hope you already have a Next js project, if not create a new Next js project with the following command.
npx create-next-app@latest my-app
I am creating a Nextjs project with app router for this tutorial but the process is similar for the older versions.
This is how my homepage looks after editing a little bit
export default function Home() {
return (
<div className="my-app text-center">
<h1 className="text-4xl my-4">React Feather Icon</h1>
<div className="mx-[48%] " >
// Puth the icon here
</div>
</div>
);
}
#Step 2: Installing react-feather
Now open a terminal inside your project and run the following command to install react-feather
icons in your project.
- npm
- yarn
npm i react-feather
#Step 3: Using feather icons
Now as we have already installed react-feather in our application, we can use it as a component in our application in the following way.
We can import the whole icon package at once
import * as Icon from 'react-feather';
export default function Home() {
return (
<div className="my-app text-center">
<h1 className="text-4xl my-4">React Feather Icon</h1>
<div className="mx-[48%] " >
<Icon.Heart />
</div>
</div>
);
}
or import specific icons from the package, the latter is best because it reduces our application size.
import { Heart } from "react-feather";
export default function Home() {
return (
<div className="my-app text-center">
<h1 className="text-4xl my-4">React Feather Icon</h1>
<div className="mx-[48%] " >
<Heart />
</div>
</div>
);
}
Output
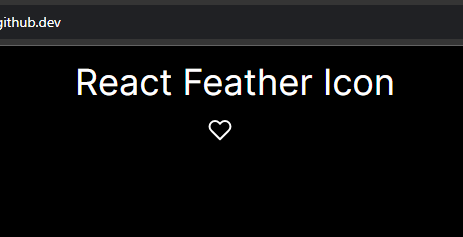
Let’s try adding some more icons
import { GitHub, Heart , Home as HomeIcon, } from "react-feather";
export default function Home() {
return (
<div className="my-app text-center">
<h1 className="text-4xl my-4">React Feather Icon</h1>
<div className="mx-[48%] flex flex-col gap-4 " >
<Heart />
<HomeIcon />
<GitHub />
</div>
</div>
);
}
Notice, that I have imported Home as HomeIcon because it has a conflicting name with my Home page component.
Output
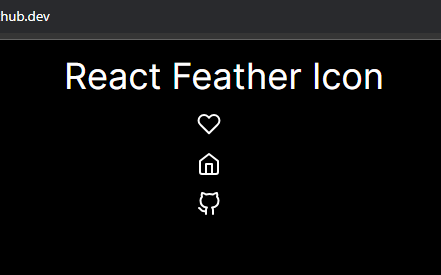
#Step 4: Customization
There are mainly these props that you can pass to customize Feather icons i.e, size
and color
.
Prop Name | Value | Funtion |
size | number (pixel) | Set the size of the icon in square |
color | string | Set the color of the icon |
strokeWidth | number | Adjust the stroke width of icon |
className | string | Pass any class name to apply custom css |
style | object | Apply inline style |
Example
import { GitHub, Heart , Home as HomeIcon, } from "react-feather";
export default function Home() {
return (
<div className="my-app text-center">
<h1 className="text-4xl my-4">React Feather Icon</h1>
<div className="mx-[48%] flex flex-col gap-4 " >
<Heart color="red" size={48} />
<HomeIcon color="skyblue" size={50} />
<GitHub size={50} />
</div>
</div>
);
}
Output
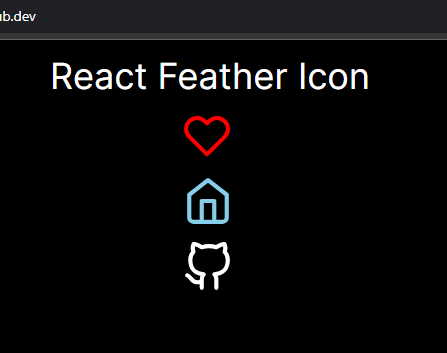
There are many additional props that you can use, to see them click on the icon component in your editor and pres ctrl+spacebar
and you’ll see the list of available props like, fill
, onClick
etc.
#Conclusion
React Feather Icons provide an excellent way to enhance the UI of your Next.js application, I hope now you know how to use them in your projects.
To explore more about Feather Icons visit the official documentation.
Thanks for visiting Tutorend please consider checking other posts too.